How to Convert JSON to CSV in Python: Step-by-Step Guide
Imad Uddin
Full Stack Developer
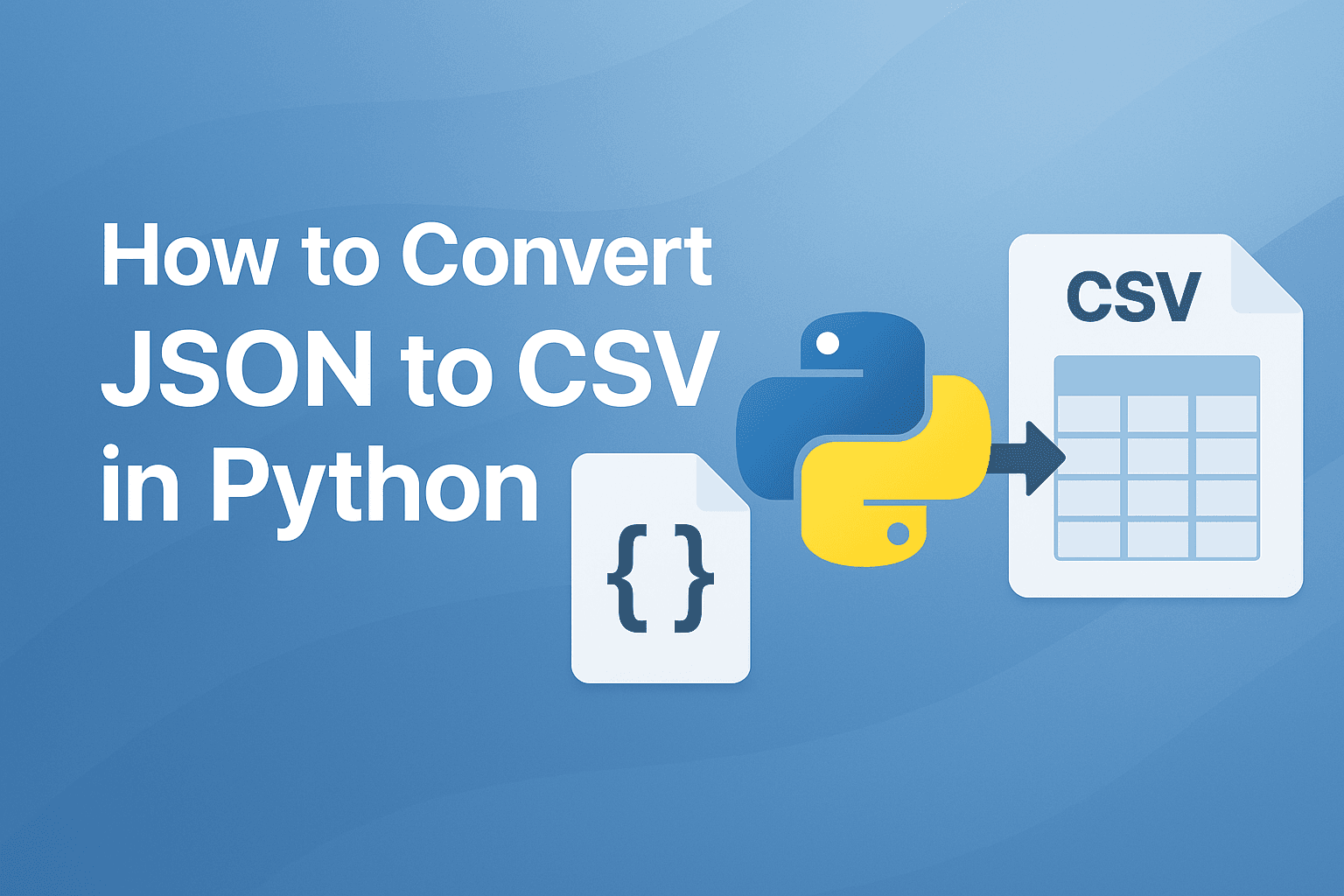
In the modern data-driven world, two formats dominate when it comes to storing and exchanging data: JSON (JavaScript Object Notation) and CSV (Comma-Separated Values). JSON is the preferred format for APIs and web applications, while CSV is widely used in spreadsheets, analytics, and databases due to its simplicity and compatibility.
If you're working with Python, converting JSON to CSV is a common task you'll face, whether you're analyzing data, integrating systems, or building data pipelines. Thankfully, Python offers powerful libraries like json, csv, and pandas that make this conversion straightforward and scalable.
This comprehensive guide will walk you through everything you need to know to convert JSON to CSV in Python β from the basics of the formats to advanced handling of nested structures. Whether youβre a beginner or an experienced developer, this guide has you covered. After reading this guide you would be able to convert JSON to CSV in python effortlessly.
π What Youβll Learn
In this in-depth guide, you'll learn:
- What JSON and CSV formats are and how they differ
- How to read and load JSON files in Python
- How to convert flat and nested JSON to CSV in Python
- The best Python libraries and tools to convert JSON to CSV
- How to automate and handle complex conversion scenarios
- Common issues and how to solve them
Letβs begin our journey into data transformation!
π Understanding JSON and CSV
What is JSON?
JSON (JavaScript Object Notation) is a lightweight, text-based format used to store and transport data. It is language-independent and commonly used for data exchange between web clients and servers.
Hereβs a simple JSON example:
Code Snippet{ "name": "Alice", "age": 30, "email": "[email protected]" }
JSON supports nested structures like arrays and objects:
Code Snippet{ "name": "Bob", "contact": { "email": "[email protected]", "phone": "1234567890" }, "skills": ["Python", "SQL"] }
What is CSV?
CSV (Comma-Separated Values) is a plain text format that represents tabular data. Each row corresponds to a record, and each column is separated by commas:
Code Snippetname,age,email Alice,30,[email protected]
CSV is widely used in business and scientific applications because it is compatible with spreadsheet programs like Excel and Google Sheets.
π§° Why Convert JSON to CSV?
While JSON is excellent for nested and complex data structures, CSV is ideal for flat, tabular data. You may need to convert JSON to CSV for:
- Data analysis and visualization
- Importing into Excel or Google Sheets
- Database ingestion
- Reporting and dashboards
- Archiving and backups
Python makes this conversion seamless and flexible.
π₯ Method 1: Convert Flat JSON to CSV Using Pythons's Built-in Libraries
Letβs start with a simple case: a flat JSON file that is easy to transform into a CSV file.
Example JSON:
Code Snippet[ { "id": 1, "name": "Alice", "email": "[email protected]" }, { "id": 2, "name": "Bob", "email": "[email protected]" } ]
Python Code:
Code Snippetimport json import csv # Load JSON data with open('data.json', 'r') as f: data = json.load(f) # Write CSV with open('output.csv', 'w', newline='') as f: writer = csv.DictWriter(f, fieldnames=data[0].keys()) writer.writeheader() writer.writerows(data)
This code reads the JSON file and writes it to CSV using csv.DictWriter, which is perfect for flat JSON arrays.
π§± Method 2: Convert Nested JSON to CSV Using Pythons's Pandas
For nested structures, the pandas library is a game changer. It provides a method called json_normalize that flattens nested dictionaries into a table.
Example Nested JSON:
Code Snippet[ { "id": 1, "name": "Alice", "contact": { "email": "[email protected]", "phone": "12345" } }, { "id": 2, "name": "Bob", "contact": { "email": "[email protected]", "phone": "67890" } } ]
Python Code:
Code Snippetimport pandas as pd import json # Load JSON with open('nested.json') as f: data = json.load(f) # Normalize and flatten df = pd.json_normalize(data) # Export to CSV df.to_csv('output.csv', index=False)
The result is a flat CSV file:
Code Snippetid,name,contact.email,contact.phone 1,Alice,[email protected],12345 2,Bob,[email protected],67890
𧬠Flatten Deeply Nested JSON
For deeply nested JSON structures, you may need to manually flatten them or recursively parse the data.
Recursive Flatten Function:
Code Snippetdef flatten_json(y): out = {} def flatten(x, name=''): if isinstance(x, dict): for a in x: flatten(x[a], name + a + '.') elif isinstance(x, list): for i, a in enumerate(x): flatten(a, name + str(i) + '.') else: out[name[:-1]] = x flatten(y) return out
You can then apply this function to each JSON object before writing to CSV. Alternatively you can use a no code free online tool JSON Flattener to make the task or flattening easier for you.
π§ͺ Handling JSON Arrays
Sometimes, JSON fields contain arrays:
Code Snippet{ "name": "Alice", "hobbies": ["reading", "cycling"] }
Convert to CSV by Joining Arrays:
Code Snippetfor item in data: item['hobbies'] = "; ".join(item.get('hobbies', []))
This joins array elements into a string before exporting to CSV.
π Automate Batch Conversion of Multiple JSON Files
Need to convert a folder full of JSON files to CSV using Python?
Code Snippetimport os import pandas as pd input_dir = 'json_files' output_dir = 'csv_outputs' os.makedirs(output_dir, exist_ok=True) for filename in os.listdir(input_dir): if filename.endswith('.json'): with open(os.path.join(input_dir, filename)) as f: data = json.load(f) df = pd.json_normalize(data) output_path = os.path.join(output_dir, filename.replace('.json', '.csv')) df.to_csv(output_path, index=False)
This script loops through JSON files and exports CSV versions automatically.
π Common Pitfalls and Solutions
Problem | Solution |
---|---|
JSONDecodeError | Check JSON syntax using online validators |
KeyError | Use .get() to safely access keys |
Inconsistent structures | Normalize the format before processing |
Nested arrays | Use loops or explode() in pandas |
Special characters in CSV | Use quoting options in csv.writer or pandas.to_csv() |
π Real-World Use Case: API to CSV in Python
Hereβs how to get JSON from an API and convert it:
Code Snippetimport requests import pandas as pd response = requests.get('https://jsonplaceholder.typicode.com/posts') data = response.json() df = pd.json_normalize(data) df.to_csv('posts.csv', index=False)
This is perfect for building data scrapers or integrating APIs into your analytics pipeline.
π‘ Best Practices
- Validate JSON before processing
- Use try-except for error handling
- Test your scripts on small datasets first
- Log each step for large batch processing
- Use descriptive filenames for output files
π FAQ: JSON to CSV in Python
How do I convert JSON to CSV using Python?
Use built-in libraries like json and csv, or pandas for more complex JSON.
Can I handle nested JSON in CSV conversion?
Yes. Use pandas.json_normalize() or write a recursive function to flatten the data.
How do I handle missing fields?
Use .get() method or pandas.fillna() to handle missing or null values gracefully.
What about converting large files?
Stream the data using ijson or chunk processing with pandas for memory efficiency.
Can I automate JSON to CSV conversion in Python?
Absolutely. Write a Python script that loops through files or processes API responses.
π Online Alternative
Donβt want to write code? Try our easy-to-use free online tool to convert JSON to CSV:
π JSON To CSV Online
It allows you to upload your JSON file and get a downloadable CSV instantly β no coding required!
π Conclusion
Whether you're a data analyst, developer, or hobbyist, converting JSON to CSV in Python is a vital skill. Python's built-in libraries and powerful tools like pandas allow you to seamlessly transform complex data into structured formats ready for analysis.
From basic one-off conversions to batch-processing pipelines, this guide covered everything you need to handle JSON-to-CSV tasks efficiently and reliably.
Get started today, and donβt forget to check out our free online tool to simplify your workflow!
Happy converting! π