How to Merge Multiple JSON Files: Step-by-Step Guide
Imad Uddin
Full Stack Developer
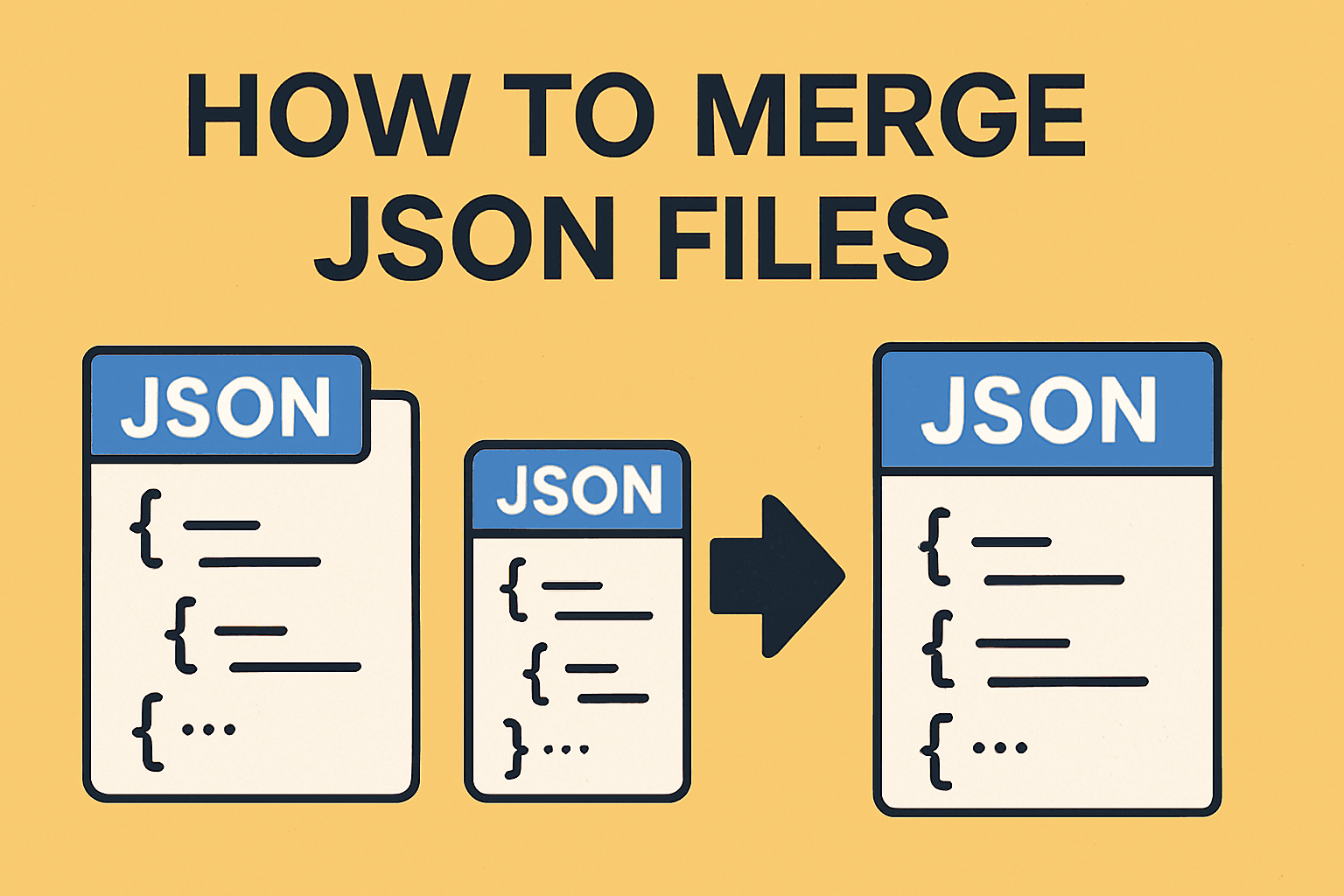
Merging multiple JSON files is a common task for developers, data engineers, and analysts. Whether you’re consolidating data exports, combining API results, or managing complex configurations, knowing how to merge JSON files efficiently can save time and prevent errors.
In this complete guide, we’ll walk you through various ways to merge multiple JSON files—from beginner-friendly online tools to advanced Python and JavaScript scripts. We’ll also cover common use cases, real-world examples, and best practices for handling large or nested JSON structures.
✅ Why Merge JSON Files?
Before diving into the methods, here’s why you might want to combine multiple JSON files into one:
- 🧩 Combine data from different sources (APIs, logs, tools)
- 📁 Unify split JSON files into a single dataset
- ⚙️ Prepare data for analysis or migration
- 📦 Bundle configuration files or test datasets
- 📊 Simplify parsing by reducing file clutter
🌐 Method 1: Use Our Online JSON Merge Tool (No Code)
If you don’t want to write any code, we’ve got you covered!
🔗 Try It Here:
✨ How It Works:
- Go to our JSON Merger Tool
- Upload two or more JSON files
- Choose your merge strategy:
- Merge arrays together
- Combine objects by key
- Preview the result
- Download the merged JSON file
🔧 Key Features:
- Works in your browser (no install)
- Supports deeply nested JSON
- Auto-validates malformed JSON
- Optional pretty print formatting
- Secure – your data never leaves your browser
🧑💻 Who It's For:
- Beginners with no coding experience
- Data analysts & non-technical users
- Quick data merge tasks on the go
🐍 Method 2: Merge JSON Files Using Python
Python is a powerful language for data processing. Here’s how to merge multiple JSON files in just a few lines.
📁 Step-by-Step: Merge JSON Files in Python
Code Snippetimport json import glob # Load all JSON files in folder json_files = glob.glob("./data/*.json") merged_data = [] for file in json_files: with open(file) as f: data = json.load(f) merged_data.extend(data) # Assumes each file contains a JSON array # Save merged file with open("merged.json", "w") as f: json.dump(merged_data, f, indent=4)
✅ Pros:
- Fully automated for many files
- Supports complex logic and validation
⚠️ Considerations:
- Assumes consistent structure (e.g., all files are arrays)
- Use try/except to catch malformed files
🌐 Method 3: Merge JSON with JavaScript (for Web Devs)
For frontend or Node.js developers, JavaScript is a natural choice.
👨💻 Browser Example (Assuming Array of Objects):
Code Snippet<input type="file" multiple id="jsonFiles" /> <script> document.getElementById("jsonFiles").addEventListener("change", async (e) => { let files = e.target.files; let merged = []; for (let file of files) { let text = await file.text(); let json = JSON.parse(text); merged = merged.concat(json); } console.log(JSON.stringify(merged, null, 2)); }); </script>
🧰 Node.js Version:
Code Snippetconst fs = require("fs"); const files = ["data1.json", "data2.json"]; let merged = []; files.forEach((file) => { const data = JSON.parse(fs.readFileSync(file)); merged = merged.concat(data); }); fs.writeFileSync("merged.json", JSON.stringify(merged, null, 2));
💻 Command-Line Approach (Linux/macOS with jq)
If you’re working on the terminal, jq can merge JSON files quickly.
Merge Array-Based Files:
Code Snippetjq -s add file1.json file2.json file3.json > merged.json
- -s = slurp multiple inputs into an array
- add merges arrays
Merge Object-Based Files:
Code Snippetjq -s 'reduce .[] as $item ({}; . * $item)' file*.json > merged.json
🧠 How to Handle Nested or Inconsistent JSON
Sometimes, not all JSON files follow the same structure.
Example:
Code Snippet// file1.json { "users": [ {"id": 1, "name": "Alice"} ] } // file2.json { "users": [ {"id": 2, "name": "Bob"} ] }
Python Merge Strategy:
Code Snippetmerged = {"users": []} for file in json_files: with open(file) as f: data = json.load(f) merged["users"].extend(data.get("users", []))
Use conditionals and .get() to safely merge partial structures.
📋 Comparison of Merge Methods
Method | Best For | Skill Level | Pros | Cons |
---|---|---|---|---|
Online Tool | Quick tasks | Beginner | No code, fast | Limited flexibility |
Python Script | Automation, large sets | Intermediate | Full control | Requires coding |
JavaScript | Web or Node.js devs | Intermediate | Client-side or backend | Manual setup |
jq CLI | DevOps/Linux | Advanced | Fast, scriptable | Syntax learning curve |
🧪 Real-World Use Cases
- ✅ Combine paginated API results into one file
- ✅ Merge config files for web apps or microservices
- ✅ Prepare datasets for training ML models
- ✅ Unify split files from backup or ETL systems
📦 Best Practices When Merging JSON Files
- ✅ Always validate input files with JSONLint
- 🧪 Use version control (e.g., Git) to track merges
- 🗂️ Backup originals before merging
- 💡 Ensure consistent structure across files
- 🧼 Sanitize duplicate or conflicting fields manually
🚀 Final Thoughts
Merging multiple JSON files doesn’t have to be overwhelming. From quick drag-and-drop tools like merge-json-files.com to flexible Python and JavaScript scripts, you now have everything you need to combine JSON data efficiently and safely.
Whether you're integrating APIs, managing logs, preparing datasets, or dealing with microservices configs—mastering JSON merging is a must-have skill for developers and analysts alike.
🧰 Try our JSON Merge Tool now—no signup, fast and free!
Happy coding & merging! 🔗💾