How to Split JSON File into Multiple Files: Step-by-Step Guide
Imad Uddin
Full Stack Developer
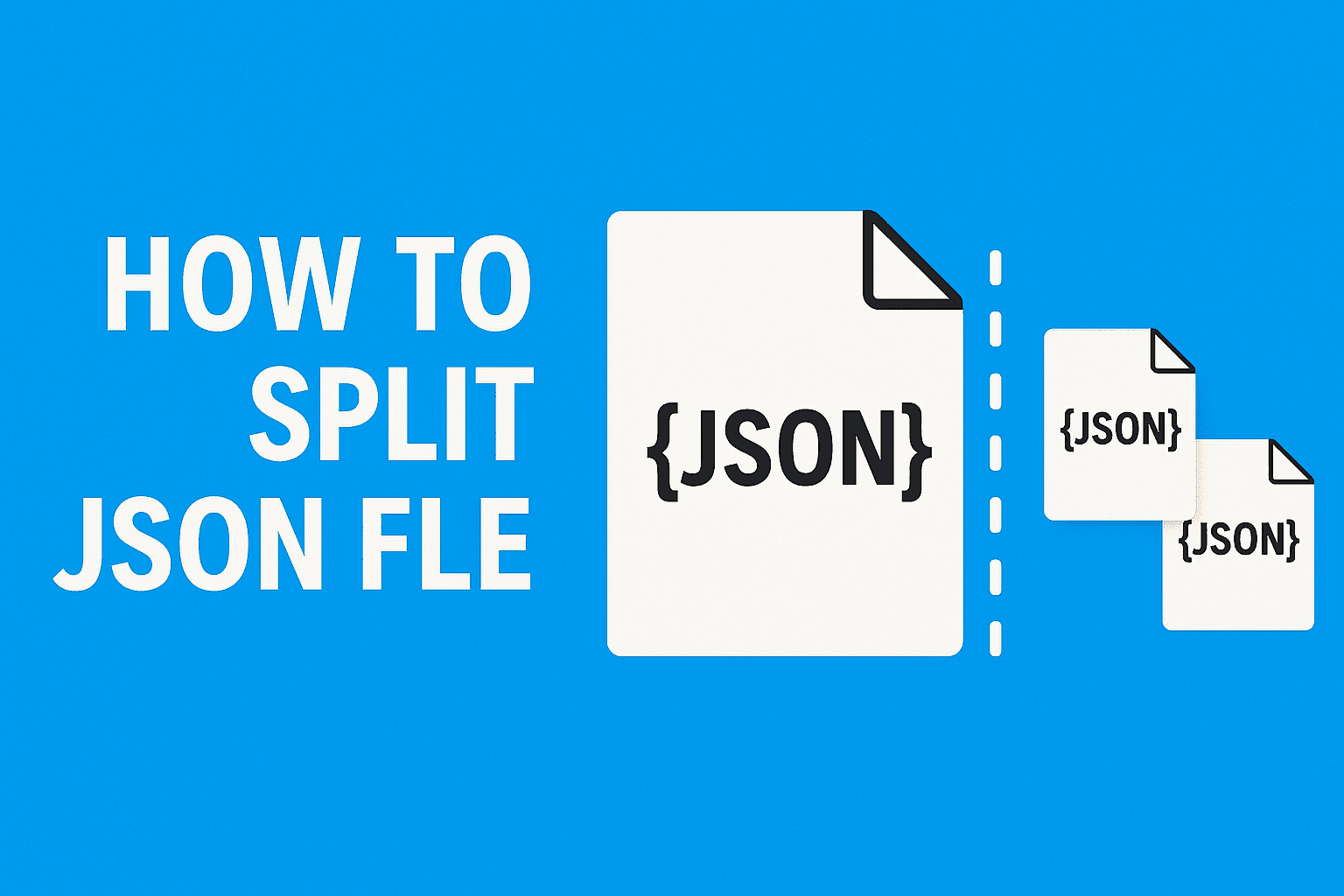
How to Split JSON File into Multiple Files: A Complete Developerβs Guide
Working with JSON files is common in web development, data processing, and software automation. But as these files grow in size, they can become difficult to manage or process efficiently. Thatβs where splitting a JSON file into multiple smaller files comes in handy.
In this comprehensive, step-by-step guide, youβll learn multiple ways to split a JSON file into multiple files using Python, online tools, command-line utilities, and more. Whether you're handling a large JSON array or separating nested JSON structures, this article has got you covered.
π Why Split a JSON File?
Before diving into the how, letβs talk about the why. Here are common reasons why developers or analysts need to split JSON files:
- Improve performance: Smaller files are faster to process
- Avoid memory issues: Some systems canβt handle massive JSON files
- Facilitate uploads: APIs or software may have file size limits
- Better organization: Separate records into logical groupings
- Easier collaboration: Distribute pieces of data among teams
- Enable version control: Smaller files make it easier to track changes
π§ JSON File Structures: What Youβre Splitting
JSON files can come in different formats:
- An array of objects
Code Snippet[ { "id": 1, "name": "Alice" }, { "id": 2, "name": "Bob" }, { "id": 3, "name": "Charlie" } ]
- Nested JSON
Code Snippet{ "users": [ { "id": 1, "name": "Alice" }, { "id": 2, "name": "Bob" } ], "metadata": { "count": 2 } }
- Line-delimited JSON (JSONL)
Code Snippet{"id": 1, "name": "Alice"} {"id": 2, "name": "Bob"}
Understanding the structure is key before you split it. JSON arrays are the most common target for splitting.
π Method 1: Split JSON File in Python
Python offers the most flexible and scalable method to split a JSON file.
Step 1: Read the JSON File
Code Snippetimport json with open('large_file.json') as f: data = json.load(f)
Step 2: Split into Chunks
Code Snippetchunk_size = 100 # records per file for i in range(0, len(data), chunk_size): chunk = data[i:i+chunk_size] with open(f'chunk_{i//chunk_size + 1}.json', 'w') as f: json.dump(chunk, f, indent=4)
This will create files like chunk_1.json, chunk_2.json, and so on.
When to Use This:
- Large array of objects
- Complete control over output format
- Automate tasks for large data pipelines
Tip:
Use argparse to turn this into a reusable CLI script:
Code Snippetimport argparse parser = argparse.ArgumentParser() parser.add_argument('input_file') parser.add_argument('--size', type=int, default=100) args = parser.parse_args()
π§° Method 2: Use Online JSON Split Tools
If you're not comfortable coding, online JSON splitters are a great alternative.
Recommended Tool:
π merge-json-files.com/json-file-splitter
How to Use:
- Upload your JSON file
- Choose the number of records per file
- Click Split
- Download the resulting files
Benefits:
- No setup required
- Beginner-friendly
- Great for quick splits
- Mobile and browser-friendly
Use Case:
Perfect for splitting exported API data or JSON dumps without installing software.
π₯οΈ Method 3: Command Line (Using jq on Linux/macOS)
For developers comfortable with the terminal, jq is a powerful command-line tool.
Install jq:
Code Snippetsudo apt install jq # Debian/Ubuntu brew install jq # macOS
Example: Split JSON Array
Code Snippetjq -c '.[]' large_file.json | split -l 100 - chunk_
This will create files chunk_aa, chunk_ab, etc., each with 100 JSON lines.
Tip:
Convert each split back to a JSON array:
Code Snippetfor file in chunk_*; do jq -s '.' "$file" > "$file.json" rm "$file" mv "$file.json" "$file" done
π Bonus: Splitting Nested JSON Structures
Letβs say your file contains deeply nested objects or grouped sections like:
Code Snippet{ "users": [...], "admins": [...] }
You can split it by key:
Code Snippetwith open('nested.json') as f: data = json.load(f) with open('users.json', 'w') as f: json.dump(data['users'], f) with open('admins.json', 'w') as f: json.dump(data['admins'], f)
This allows you to isolate and split different sections of a nested JSON structure easily.
π§ͺ Real-World Use Case: JSON API Pagination
Many APIs return paginated results. Hereβs a simple way to split them as you collect:
Code Snippetimport requests all_data = [] for page in range(1, 6): response = requests.get(f'https://api.example.com/data?page={page}') all_data.extend(response.json()) with open('combined.json', 'w') as f: json.dump(all_data, f)
Then use the Python split technique to divide the result.
π§ Tools Comparison Table
Method | Best For | Technical Skill | Pros | Cons |
---|---|---|---|---|
Python Script | Automation, full control | Intermediate/Advanced | Scalable, customizable | Requires coding |
Online Tool | Quick tasks | Beginner | No install, user-friendly | Limited flexibility |
jq (CLI) | Linux users, large files | Intermediate | Fast, terminal native | Learning curve |
β Common Errors When Splitting JSON Files & Solutions
Error | Likely Cause | Fix |
---|---|---|
JSONDecodeError | Malformed input file | Validate JSON using jsonlint.com |
TypeError: list indices must be integers | Accessing a list like a dict | Check your data structure |
Permission Error | Writing to protected directory | Change file path or use sudo |
Out of Memory | Very large input JSON | Stream read or increase RAM |
π§ Best Practices When Splitting JSON Files
- Always validate the JSON before and after splitting
- Keep backup copies of your original files
- Use descriptive filenames like users_part_1.json
- Add logging to Python scripts for error handling
- If splitting for upload, check the file size limit of your platform
- Consider compressing large sets using .zip or .tar.gz
π Recap: Ways to Split JSON Files
- π Python: Best for automation, flexible
- π Online Tools: Great for non-coders
- π» jq CLI: Efficient for large file processing
Choose the method that fits your project and technical comfort level.
π Final Thoughts
Whether you're a developer, data engineer, or analyst, learning how to split a JSON file into multiple files can save time and prevent headaches. From automation scripts in Python to fast online tools and robust CLI utilities, you now have the knowledge and tools to handle even the largest JSON datasets with ease.
π Try our free tool: merge-json-files.com/json-file-splitter for fast, no-code splitting.
If you're working on batch processing, API integrations, or database imports, mastering JSON file splitting is a vital skill. Use this guide as a reference whenever you need to streamline your JSON workflows.
Happy JSON splitting! π₯π